Salesforce provides a way to fetch external data using Salesforce Apex callout. The Apex code uses the Remote Site Settings URL to retrieve the data from an endpoint, and also the Apex integrates with Web services by using SOAP, WSDL, and HTTP (REST).
The example below shows how to display the external data in Salesforce from an endpoint using Apex callouts.
Step 1: Create a Remote Site Settings.
URL (https://raw.githubusercontent.com)
The URL contains the candidate details in JSON format with a few fields: Candidate name, Account number, Source, Website, and Email.
Step 2: Create a Wrapper Class
Consolewrap.apxc
public class consolewrap { public String Name{get;set;} public String AccNumber{get;set;} public String Source{get;set;} public String Site{get;set;} public String Email{get;set;} }
The Consolewrap wrapper class contains variables to hold the values from the external data source.
Step 3: Create a Visualforce page
Callout.vfp
<apex:page controller="calloutcontroller" title="JSON table" > <apex:form > <apex:pageBlock > <apex:pageBlockTable value="{!performcallout}" var="wrap" width="100%"> <apex:column headerValue="Name" value="{!wrap.Name}"/> <apex:column headerValue="AccountNumber" value="{!wrap.AccNumber}"/> <apex:column headerValue="Source" value="{!wrap.Source}"/> <apex:column headerValue="Website" value="{!wrap.Site}"/> <apex:column headerValue="Email" value="{!wrap.Email}"/> </apex:pageBlockTable> </apex:pageBlock> </apex:form> </apex:page>
The VF page contains the PageBlockTable to display the candidate details in a table format by calling the Controller class.
Step 4: Create an Apex Class
Calloutcontroller.apxc
public class Calloutcontroller { public List<consolewrap> ConsoleWrapperList{get;set;} public List<consolewrap> getperformcallout(){ ConsoleWrapperList = new List<consolewrap>(); HttpRequest req = new HttpRequest(); HttpResponse res = new HttpResponse(); Http http = new Http(); req.setEndpoint ('https://raw.githubusercontent.com/ parthiban019/samplejson/master/ student.json'); req.setMethod('GET'); res = http.send(req); if(res.getstatusCode() == 200 && res.getbody() != null){ ConsoleWrapperList=(List<consolewrap>) json.deserialize(res.getbody(), List<consolewrap>.class); } return consolewrapperlist; } }
The Calloutcontroller class uses the HTTP request and GET method to retrieve the data from the endpoint which is in JSON format and the class populates the external data in a wrapper list used to display the data on the Visualforce page.
Step 5: Execute the Visualforce page
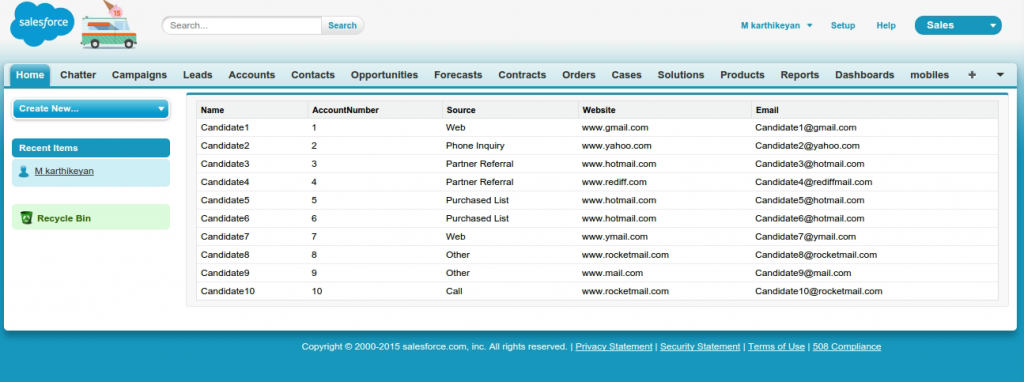
Now, the Visualforce page displays the data from the external system.
Reference Link: Salesforce: Apex Web Services and Callouts