Introduction:
A wrapper or container class is a class, a data structure, or an abstract data type which contains different objects or collection of objects as its members.
A wrapper class is a custom object where the wrapper class properties are defined. Consider a custom object in Salesforce. It contains different fields of different data types. Similarly, wrapper class is a custom class which has different data types or properties. As per requirement, we can wrap different object types or any other types in a wrapper class.
The Example below shows how to display the multi-select picklists using the wrapper class.
Step 1: Create a Visualforce page
multiselect.vfp
<apex:page controller="multiselect" > <apex:form> <apex:outputPanel id="out"> <center><apex:outputText value="Wrapper class with multi-select picklists" style="font-style:normal;font-weight:bold;font-size:18px;text-align:center;width :100%;"> </apex:outputText></center> <br/> <table border="1" style="position: relative;left:37%;"> <apex:repeat value="{!Lists}" var="l"> <tr> <td> <apex:selectList value="{!l.countries}" multiselect="true"> <apex:selectOptions value="{!l.items}"/> </apex:selectList><p/> </td> <td> <apex:actionstatus id="status" startText="testing..."> <apex:facet name="stop"> <apex:outputPanel> <p>You have selected:</p> <apex:dataList value="{!l.countries}" var="c">{!c}</apex:dataList> </apex:outputPanel> </apex:facet> </apex:actionstatus> </td> </tr> </apex:repeat> </table> </apex:outputPanel> <br/> <center><apex:commandButton value="Submit" action="{!test}" status="status"/></center> </apex:form> </apex:page>
Step 2: Create a Controller class for the Visualforce page
public class multiselect{ public List<selectList> lists {get; set;} public multiselect() { lists = new List<selectList>(); for (Integer i = 0; i < 3; i++) { lists.add(new selectList(i)); } } public PageReference test() { return null; } public class selectList { Integer intValue; String[] countries = new String[]{}; public selectList(Integer intValue) { this.intValue = intValue; } public List<SelectOption> getItems() { List<SelectOption> options = new List<SelectOption>(); options.add(new SelectOption('Value:' + intValue + ' - US','Label:' + intValue + ' - US')); options.add(new SelectOption('Value:' + intValue + ' - CANADA','Label:' + intValue + ' - Canada')); options.add(new SelectOption('Value:' + intValue + ' - MEXICO','Label:' + intValue + ' - Mexico')); return options; } public String[] getCountries() { return countries; } public void setCountries(String[] countries) { this.countries = countries; } } }
In this controller apex class, we have used a wrapper class selectList in which we define the multi-select picklist values by using the getter and setter methods.
Step 3: Execute the Visualforce page
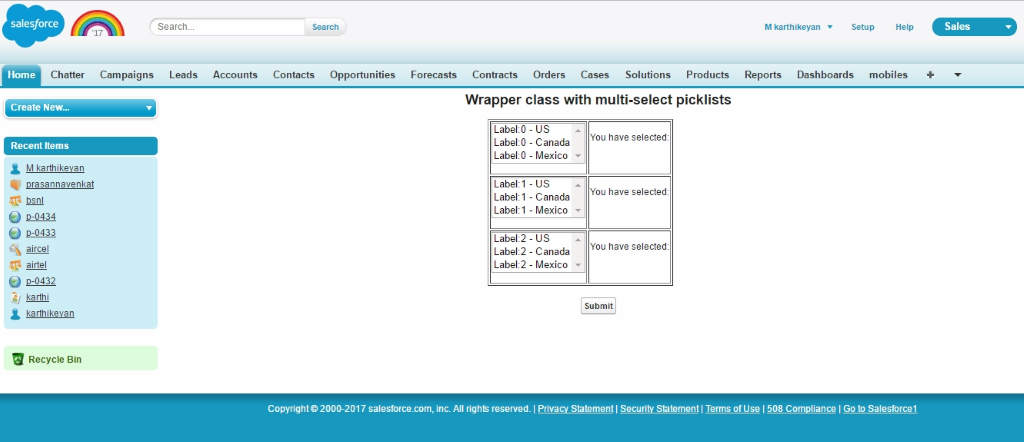
The Visualforce page displays a table with multi-select picklist field in the first column, and if one or more values are selected, the selected values will be displayed in the second column.
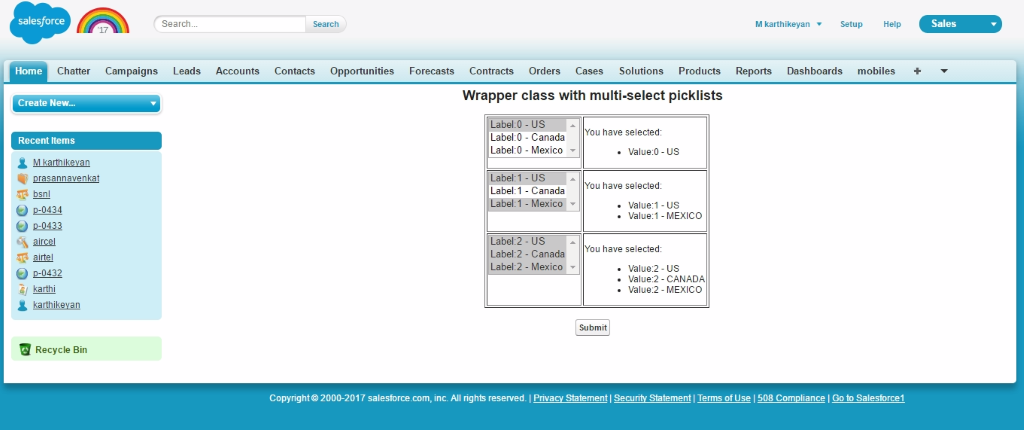
Reference Link: Wrapper class